Supercharging SveltePak: Integrating Supabase with Prisma
August 14, 2024
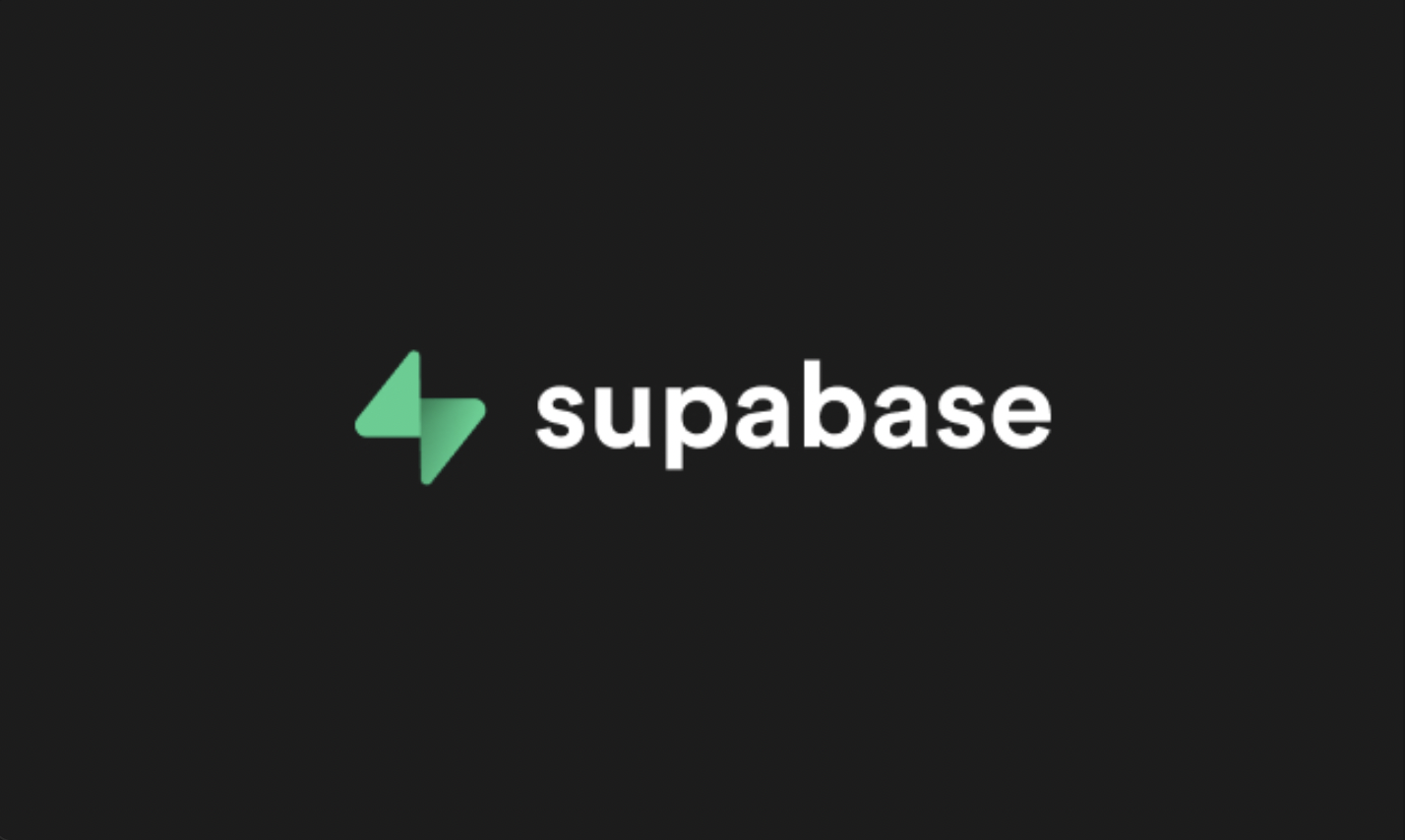
Supercharging SveltePak: Integrating Supabase with Prisma
In the world of modern web development, choosing the right tools can significantly impact your project’s success. Today, we’re exploring how to enhance your SveltePak projects by integrating Supabase with Prisma. This powerful combination brings together the best of both worlds: Supabase’s robust backend-as-a-service features and Prisma’s type-safe database toolkit.
Why Combine Supabase and Prisma?
Before we dive into the how-to, let’s understand why this integration is beneficial:
- Supabase: Offers a full suite of backend services including authentication, real-time subscriptions, and file storage.
- Prisma: Provides a type-safe database toolkit that simplifies database workflows.
- Together: They offer a comprehensive, scalable, and developer-friendly backend solution.
Setting Up Supabase and Prisma in SveltePak
SveltePak already comes with Prisma configured. Here’s how to add Supabase to the mix:
Install Supabase client:
npm install @supabase/supabase-js
Create a Supabase project and get your API keys from the Supabase dashboard.
Add Supabase configuration to your .env
file:
SUPABASE_URL=your_supabase_project_url
SUPABASE_ANON_KEY=your_supabase_anon_key
Create a Supabase client in src/lib/supabase.ts
:
import { createClient } from "@supabase/supabase-js";
const supabaseUrl = import.meta.env.VITE_SUPABASE_URL;
const supabaseAnonKey = import.meta.env.VITE_SUPABASE_ANON_KEY;
export const supabase = createClient(supabaseUrl, supabaseAnonKey);
Integrating Supabase with Prisma
Now that we have both Supabase and Prisma set up, let’s integrate them:
Use Supabase as the database provider for Prisma. Update your prisma/schema.prisma
:
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
Set your DATABASE_URL
in .env
to point to your Supabase PostgreSQL connection string.
Run Prisma migration:
npx prisma migrate dev
Leveraging Supabase and Prisma in Your SveltePak Project
Here’s an example of how you might use both Supabase and Prisma in a SveltePak route:
import { supabase } from "$lib/supabase";
import { prisma } from "$lib/prisma";
import type { RequestHandler } from "./$types";
export const POST: RequestHandler = async ({ request }) => {
// Supabase authentication
const {
data: { user },
} = await supabase.auth.getUser();
if (!user) {
return new Response(JSON.stringify({ error: "Unauthorized" }), {
status: 401,
});
}
// Get data from request
const { title, content } = await request.json();
// Use Prisma to create a new post
const post = await prisma.post.create({
data: {
title,
content,
authorId: user.id,
},
});
return new Response(JSON.stringify(post), { status: 201 });
};
In this example, we’re using Supabase for authentication and Prisma for database operations.
Advanced Tips
Real-time Updates: Use Supabase’s real-time features with Prisma:
supabase
.channel("custom-all-channel")
.on(
"postgres_changes",
{ event: "*", schema: "public", table: "posts" },
async (payload) => {
// Fetch updated data using Prisma
const updatedPost = await prisma.post.findUnique({
where: { id: payload.new.id },
});
// Update your Svelte store or component state
}
)
.subscribe();
File Storage: Use Supabase for file storage and Prisma for metadata:
// Upload file to Supabase storage
const { data, error } = await supabase.storage
.from('avatars')
.upload(`avatar-${userId}.png`, file);
if (data) {
// Store file metadata using Prisma
await prisma.user.update({
where: { id: userId },
data: { avatarUrl: data.path }
});
}
Transactions: Use Prisma's transactions for complex operations:
typescriptCopyconst result = await prisma.$transaction(async (prisma) => {
const user = await prisma.user.create({ ... });
const post = await prisma.post.create({ ... });
return { user, post };
});
Conclusion
Integrating Supabase with Prisma in your SveltePak project provides a powerful, flexible, and developer-friendly backend solution. You get the best of both worlds: Supabase’s comprehensive backend-as-a-service features and Prisma’s type-safe database operations. This combination allows you to:
- Rapidly develop and prototype with Supabase’s instant backend setup
- Ensure type safety and improve developer experience with Prisma
- Scale your application with confidence, leveraging both tools’ strengths
By following the steps and examples in this guide, you’re well on your way to creating robust, scalable applications with SveltePak, enhanced by the Supabase-Prisma powerhouse. Remember, SveltePak is designed to be flexible and adaptable to your needs. This Supabase-Prisma integration is just one of many ways you can extend and customize your SveltePak projects to fit your unique requirements.
Happy coding!
Ready to supercharge your SveltePak project with Supabase and Prisma? Get started with SveltePak today and take your development to the next level!