Mastering Svelte's Lifecycle Functions: A Step-by-Step Guide
April 15, 2024
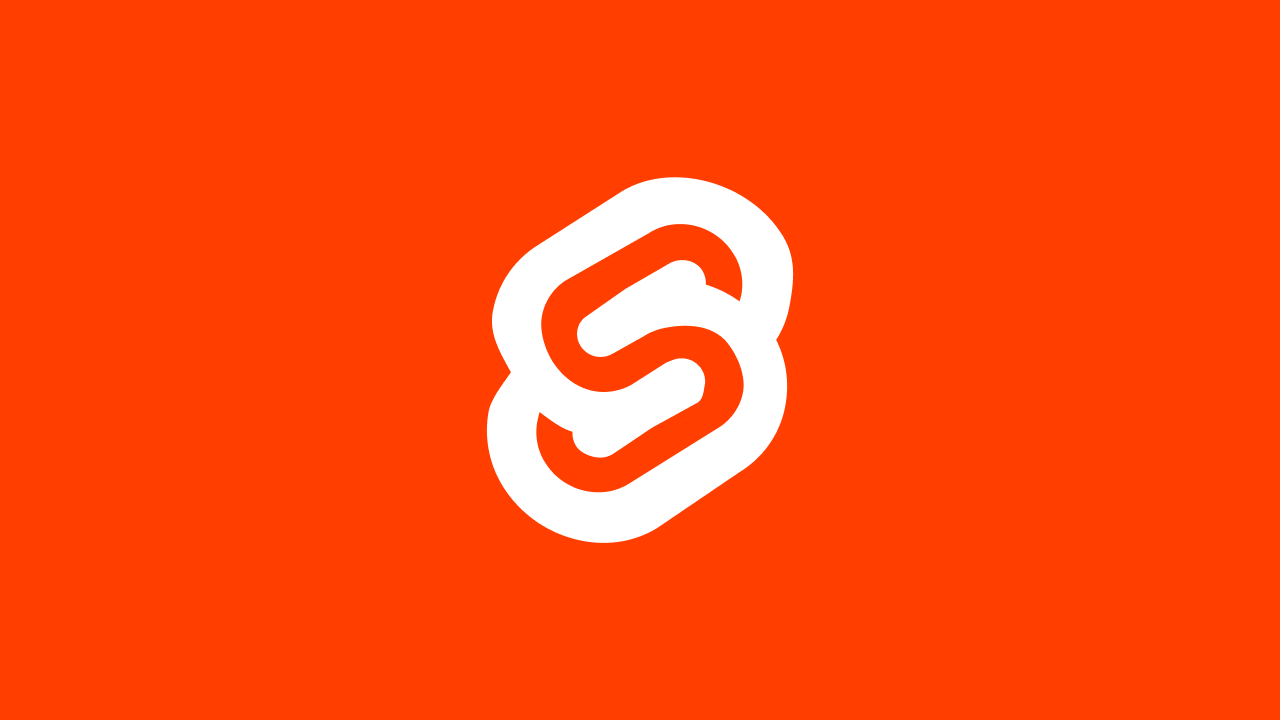
Mastering Svelte’s Lifecycle Functions: A Step-by-Step Guide
Svelte is a powerful component framework that has been gaining a lot of traction in the web development community. One of the key features that sets Svelte apart is its unique approach to handling component lifecycle events. In this article, we’ll dive deep into Svelte’s lifecycle functions and explore how you can leverage them to build more robust and dynamic web applications.
Understanding Svelte Lifecycle Functions
In Svelte, each component goes through a series of lifecycle stages, and for each of these stages, Svelte provides a corresponding lifecycle function. These functions allow you to hook into the component’s lifecycle and perform specific actions at different points in the component’s existence.
The main Svelte lifecycle functions are:
onMount
: Called after the component is first rendered to the DOM.onDestroy
: Called just before the component is destroyed.onUpdate
: Called after the component has been updated (including the first render).onContext
: Called when a context value changes.beforeUpdate
: Called before the component is updated.afterUpdate
: Called after the component has been updated.
Using onMount to Fetch Data
One of the most common use cases for the onMount lifecycle function is fetching data from an API or external source. Let’s look at an example:
import { onMount } from "svelte"
;
let data = [];
onMount(async () => {
try {
const response = await fetch("https://api.example.com/data");
data = await response.json();
} catch (error) {
console.error("Error fetching data:", error);
}
});
In this example, we’re using the onMount function to fetch data from an API endpoint as soon as the component is rendered to the DOM. The fetched data is then stored in the data variable, which can be used to render the component’s UI.
Cleaning Up with onDestroy
Another important lifecycle function is onDestroy, which is called just before the component is destroyed. This is useful for cleaning up any resources or subscriptions that your component might have created, such as event listeners or timers.
Let’s look at an example of using onDestroy to clean up an event listener:
import { onMount, onDestroy } from "svelte";
let element;
onMount(() => {
const handleClick = () => {
console.log("Clicked");
};
element.addEventListener("click", handleClick);
return () => {
element.removeEventListener("click", handleClick);
};
});
In this example, we’re adding a click event listener to a DOM element in the onMount function. However, to ensure that we don’t have any memory leaks, we’re removing the event listener in the onDestroy function, which is called just before the component is destroyed.
Reacting to Updates with onUpdate
The onUpdate function is called after the component has been updated, including the first render. This is useful for responding to changes in the component’s state or props, and can be used to trigger side effects or update the UI.
Let’s look at an example of using onUpdate to update the page title whenever a component’s prop changes:
export let pageTitle = "My Page";
onMount(() => {
document.title = pageTitle;
});
onUpdate(() => {
document.title = pageTitle;
});
In this example, we’re using the onMount function to set the initial page title, and the onUpdate function to update the page title whenever the pageTitle prop changes.
Conclusion
Svelte’s lifecycle functions are a powerful tool for building dynamic, responsive web applications. By understanding how to use these functions effectively, you can create components that are easy to maintain, testable, and efficient.
In this guide, we’ve explored three of the most commonly used Svelte lifecycle functions: onMount, onDestroy, and onUpdate. By using these functions to fetch data, clean up resources, and respond to updates, you can create Svelte components that are both powerful and easy to reason about.
As you continue to build with Svelte, be sure to explore the other lifecycle functions and experiment with different use cases. With a solid understanding of Svelte’s lifecycle management, you’ll be well on your way to creating exceptional web experiences.